You can use the interface methods to enable an app to trigger certain actions on the Freshteam user interface.
showModal
The method opens a modal dialog box in an IFrame to display HTML content to users. Events methods and interface methods are not supported within the Modal IFrame.
1. In the following code, the template field is mandatory. If no value is specified, an error message is displayed when the method is run.
2. The title field is optional and supports 35 characters; beyond that the title is truncated. The default title is Modal.
Copied Copy
1 2 3 4 5 6 7 8 | client.interface.trigger("showModal", { title: "Sample Modal", template: "modal.html" }).then(function(data) { // data - success message }).catch(function(error) { // error - error object }); |
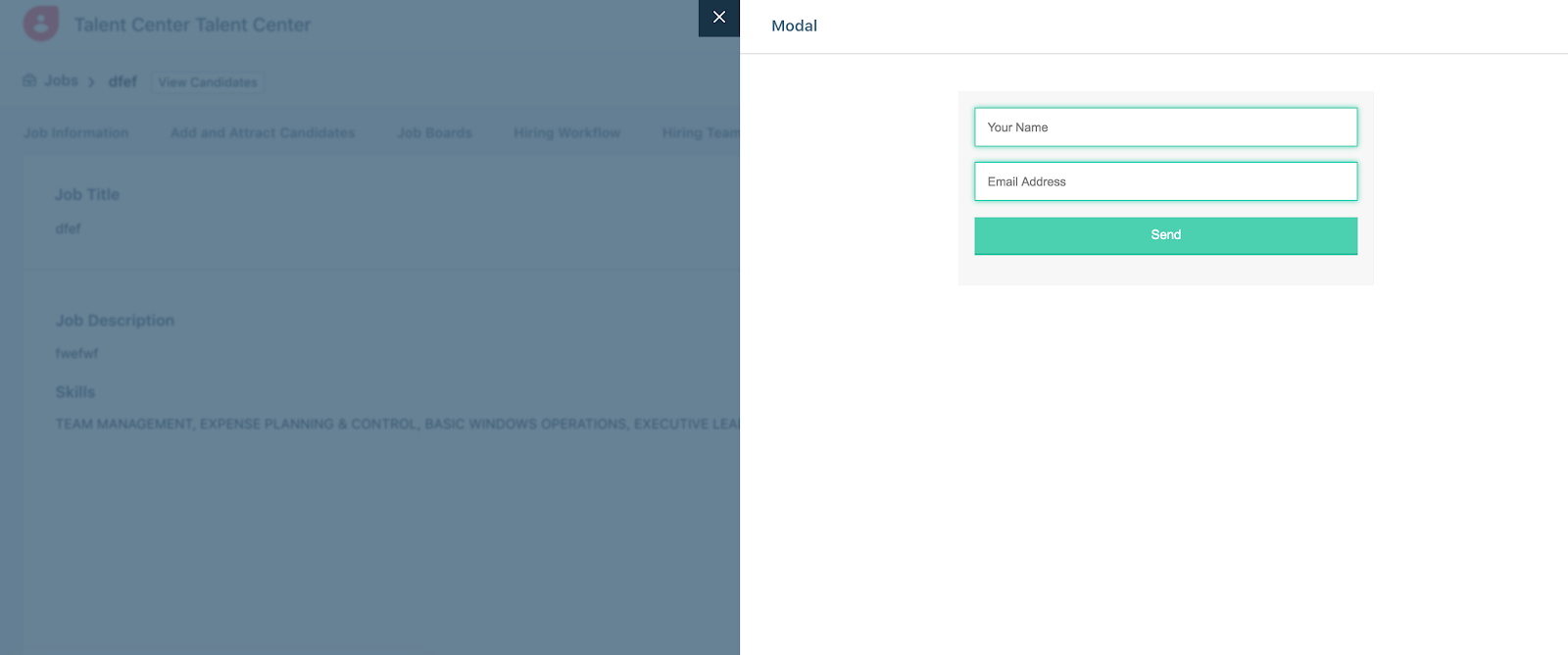
showDialog
The method displays a dialog box, with the title specified, based on a specified template. The dialog box is displayed in an IFrame.
Notes:
1. In the following code, the template field is mandatory. If no value is specified, an error message is displayed when the method is run. The template field value specifies the path to the template that is used to render the dialog box.
2. The title field supports 30 characters; beyond that the title is truncated. If the title parameter is omitted, the default title is Show Dialog. If title is “ ”, the dialog box is displayed without a title.
Sample template.html
Copied Copy1 2 3 4 | client.interface.trigger("showDialog", { title: "Sample Dialog", template: "filename.html" }); |
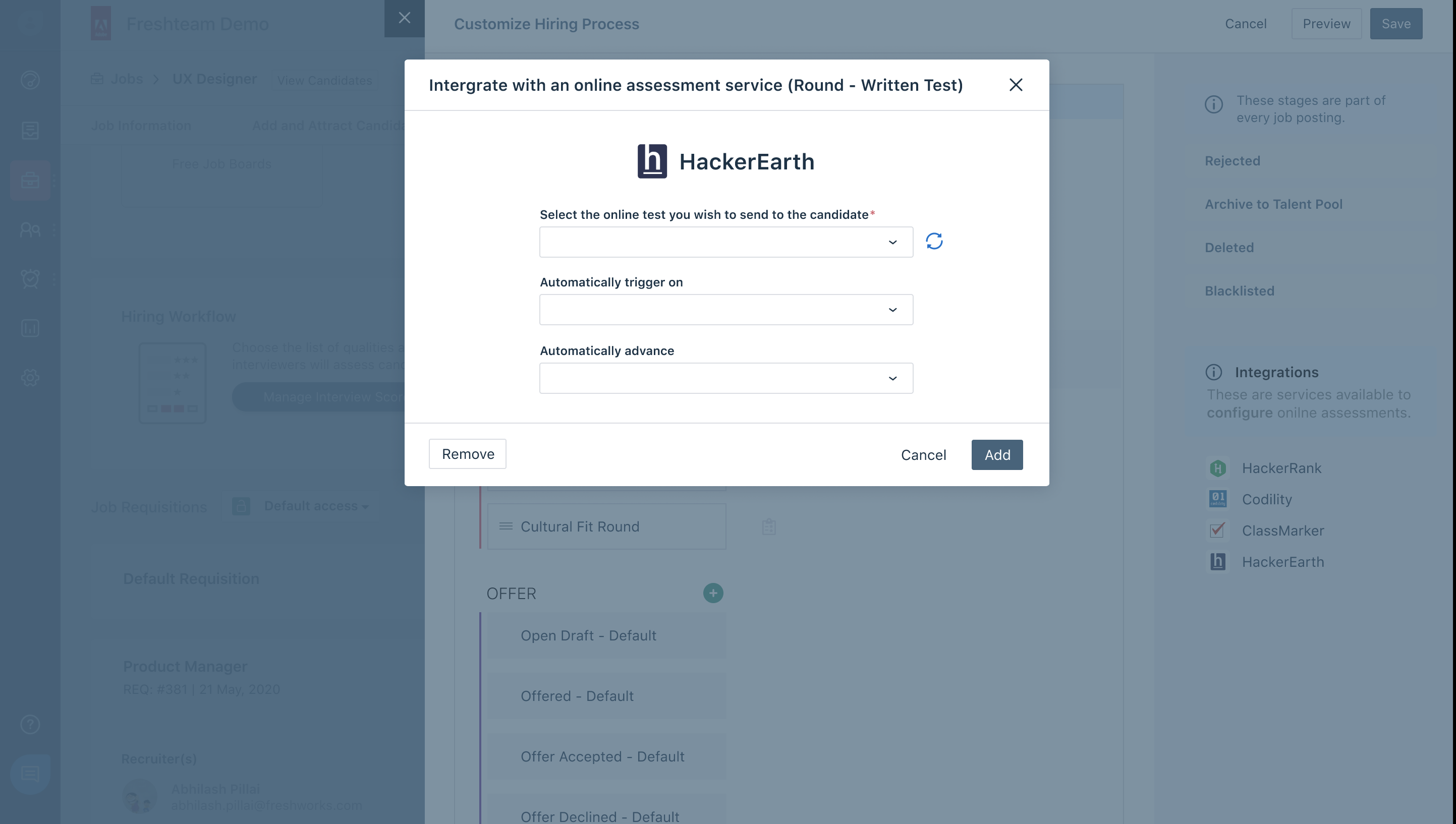
showConfirm
The method displays a confirmation dialog box to users with a message and the save and cancel buttons. The message and labels corresponding the save and cancel buttons can be configured as shown in the following code. By default, the dialog box displays the Save and Cancel buttons.
Timeout for the confirmation dialog box is 10 seconds.
1. In the following code, the message field is mandatory and supports a maximum of 100 characters; beyond that the message is truncated.
2. The saveLabel and cancelLabel fields support a maximum of 11 characters.
Sample Confirmation Dialog Box with Default Buttons
Copied Copy1 2 3 4 5 6 7 8 9 | client.interface.trigger("showConfirm", { message: "Do you want to save the changes?", saveLabel: "Save", cancelLabel: "Cancel" }).then(function(result) { // data - success message }).catch(function(error) { // error - error object; }); |
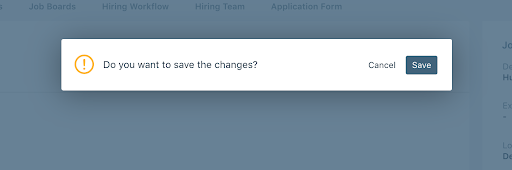
showNotify
The method displays an alert notification on the user interface.
Notes:
1. In the following code, the message field is mandatory and supports a maximum of 100 characters; beyond that the message is truncated.
2. The type field is mandatory and the supported values are: success, info, warning, and danger.
Sample Alert Notification
Copied Copy1 2 3 4 5 6 7 8 | client.interface.trigger("showNotify", { type: "success", message: "Saved changes successfully" }).then(function(data) { // data - success message }).catch(function(error) { // error - error object }); |
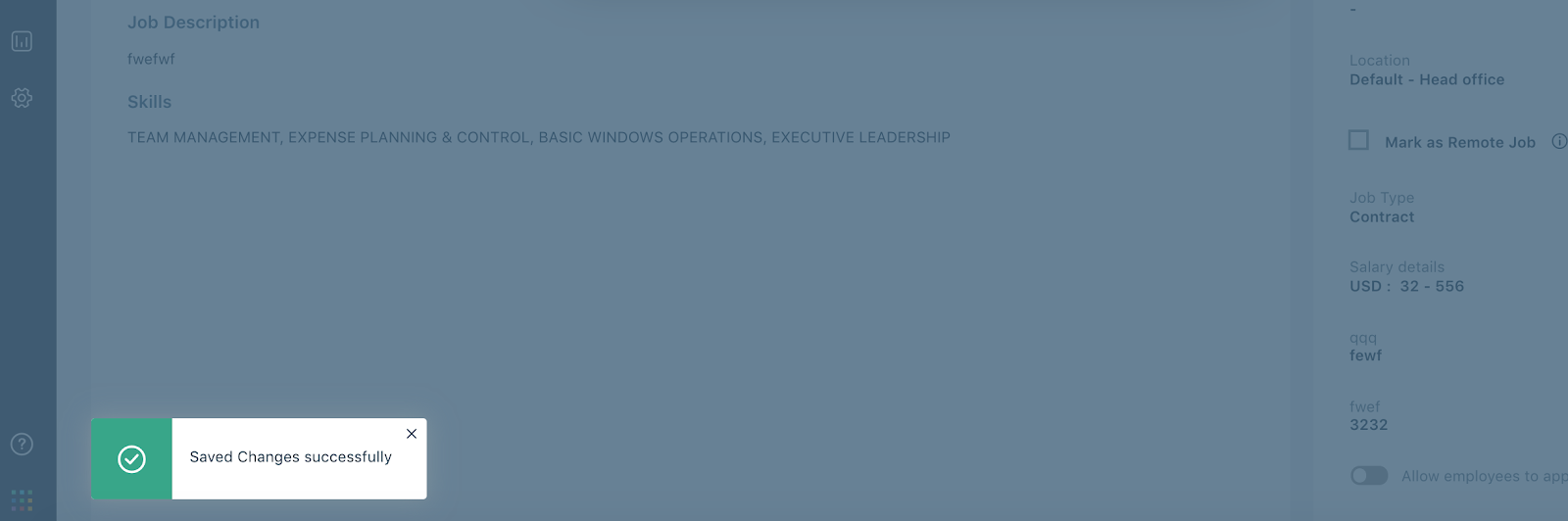
openCandidateEmailEditor
The method opens the candidate email editor and pre-populates the subject, body, recipients’ email addresses, and sets a flag (private) to indicate if subsequent response from the candidate is visible to the entire hiring team or only specific people.
Sample app.js
Copied Copy1 2 3 4 5 6 7 8 9 10 | client.interface.trigger("openCandidateEmailEditor", { subject: "Subject inserted", body: "<html content>", cc: ["sam@robocorp.com" ,"anna@robocorp.com" ], private: false }).then(function(data) { // data - success message }).catch(function(error) { // error - error object }); |
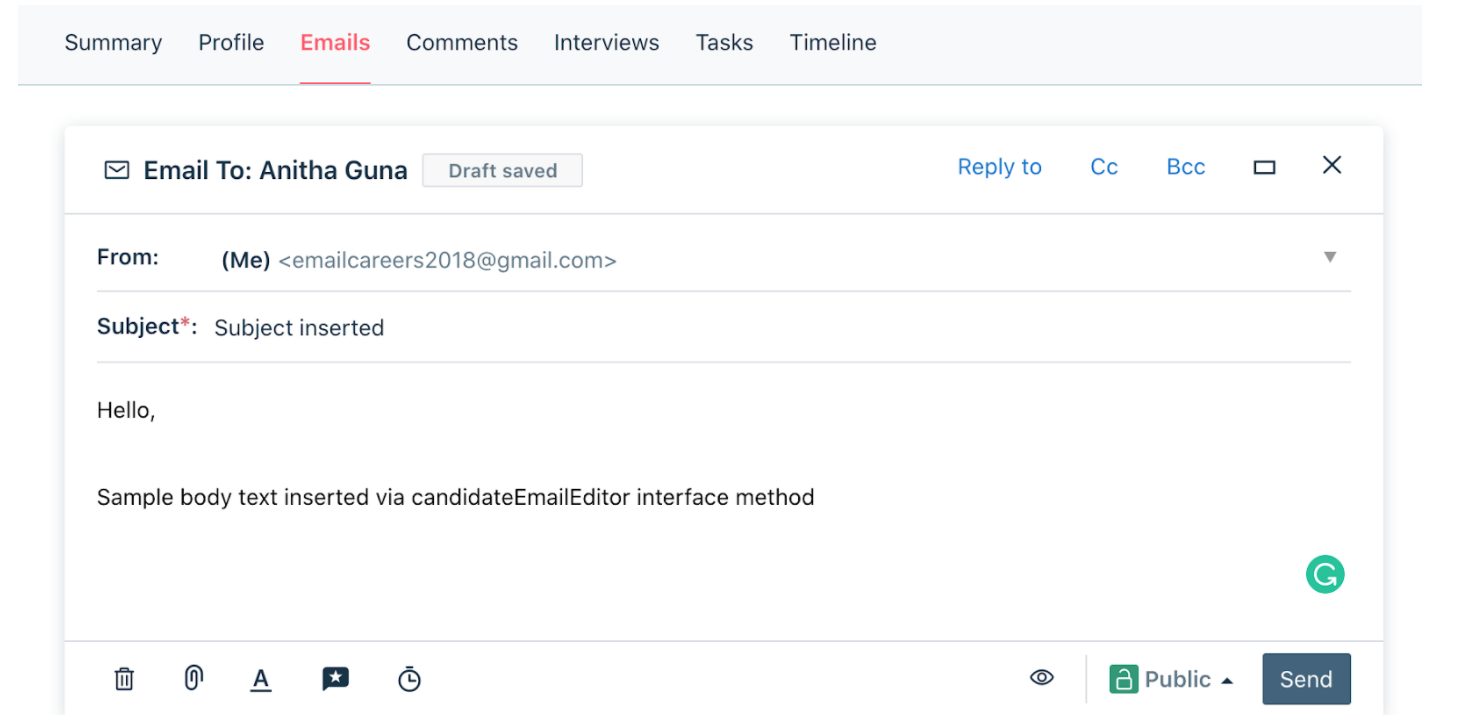