You can use these methods to enable apps to react to events that occur in the user interface of a page. Events include button clicks, changes, and updates to field values. You can configure event listeners in the app.js file. When an event occurs, the corresponding event listener invokes an event callback method and passes a standard payload to the method.
Configure events
To configure a click or change event listener and the corresponding event callback method:
- From your app’s root directory, navigate to the app.js file.
- Enter the event name, corresponding callback method, and callback method definition as follows:
1 2 3 4 5 6 | var eventCallbackMethod = function (event) { //Callback method definition - actions to perform //event payload consists of a type attribute that identifies the event console.log(event.type + " event occurred"); }; client.events.on("eventName", eventCallbackMethod); |
Possible eventName values: candidates.onEmailSent, jobPosting.onStatusChange
Payload attributes
The event payload that is passed to the callback method contains a type attribute that specifies the event name and a data object. The data object can be accessed through the event.helper.getData() method as follows:
Copied Copy1 2 | // event_data is a JSON whose value depends on the event var event_data = event.helper.getData(); |
For click events, the event.helper.getData() method returns an empty JSON object. For change events, the event.helper.getData() method returns an object of the following format:
Copied Copy1 2 3 4 | { "old": "original value of the field"; "new": "modified value of the field" } |
Click events
The events occur when an app user clicks a button or a link of the Freshteam user interface.
candidates.onEmailSent - The event is triggered when an app user clicks the Send button in the Candidate details page > Emails > Send Email editor.
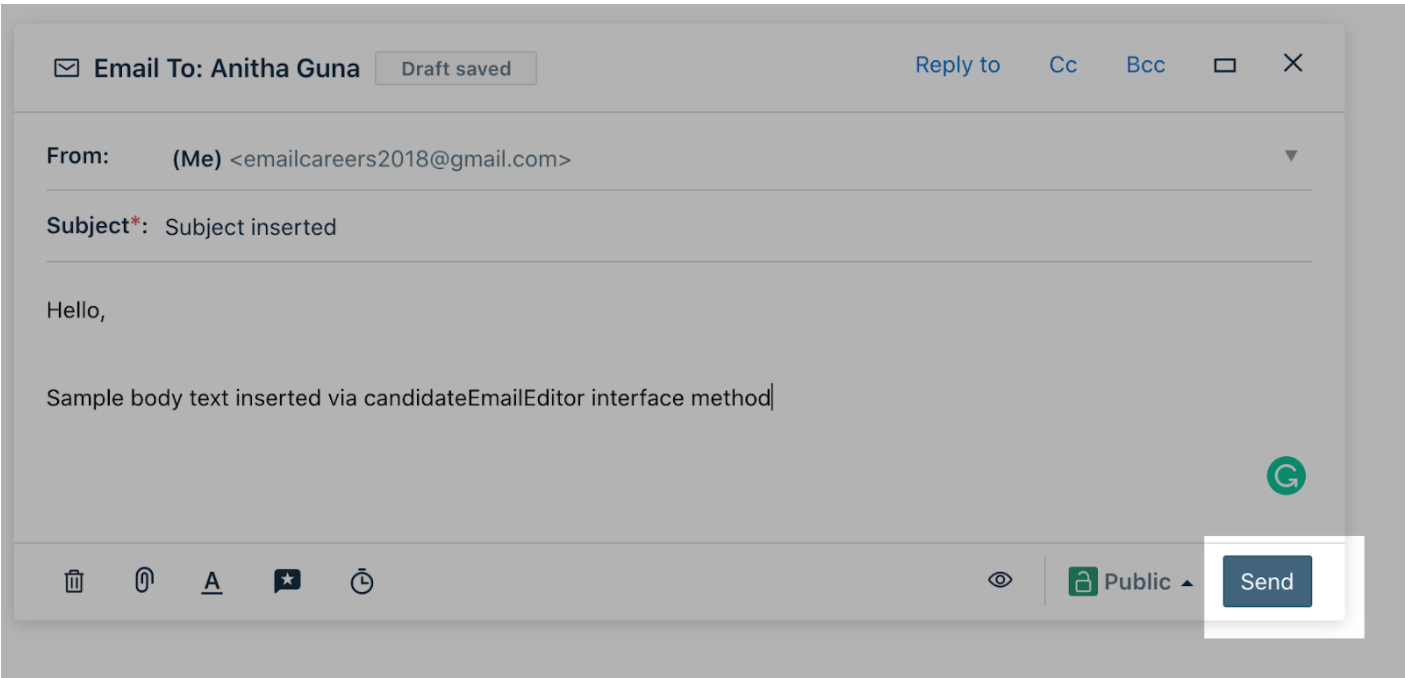
1 2 3 4 | var onEmailSentMethod = function (event) { console.log(event.type + " event occurred"); }; client.events.on("candidates.onEmailSent ", onEmailSentMethod); |
Change events
The events occur when there is a change to a value on the Freshteam user interface.
jobPosting.onStatusChange - The event is triggered when there is a change to the job status on the Job Details page.
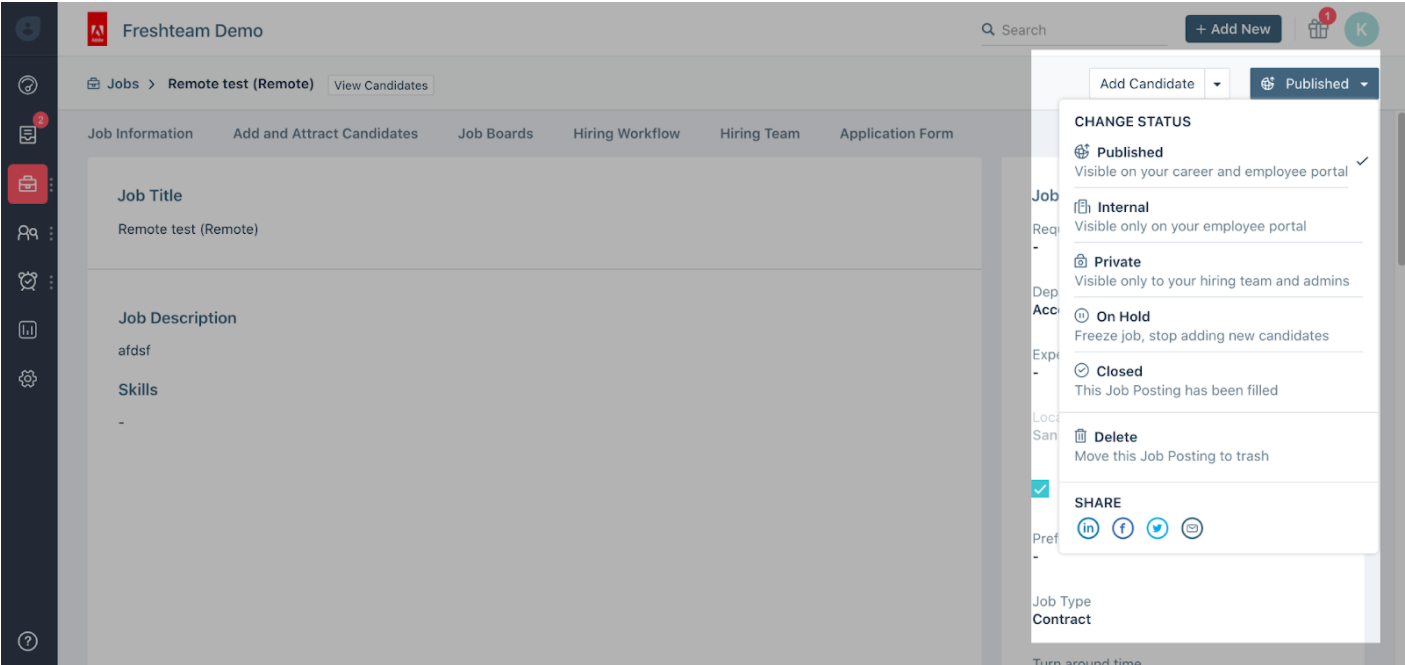
1 2 3 4 5 | var OnJobStatusChangeMethod = function (event) { var data = event.helper.getData(); console.log(event.type + "changed" + data.old + "to" + data.new); }; client.events.on("jobPosting.onStatusChange", OnJobStatusChangeMethod); |